System.security.cryptography.aes Generate Random Key
- Steam
- Data Security And Cryptography
- Cryptography In Information Security
- System Security Cryptography Algorithms
- System.security.cryptography.aes Generate Random Key Free
The Crypto.getRandomValues()
method lets you get cryptographically strong random values. The array given as the parameter is filled with random numbers (random in its cryptographic meaning).
To guarantee enough performance, implementations are not using a truly random number generator, but they are using a pseudo-random number generator seeded with a value with enough entropy. The PRNG used differs from one implementation to the other but is suitable for cryptographic usages. Implementations are also required to use a seed with enough entropy, like a system-level entropy source.
Here are the examples of the csharp api class System.Security.Cryptography.Aes.Create taken from open source projects. By voting up you can indicate which examples are most useful and appropriate. Random Key Generator for Passwords, Encryption Keys, WPA Keys, WEP Keys, CodeIgniter Keys, Laravel Keys, and much more. Don't got what you're looking for! Send us a mail or contribute on Github. KeyGen.io - Random Key Generators. Decent Password. Strong Password. Very Strong Password. CodeIgniter Encryption Key. AES encryption/decryption using pure.NET and streams. Ask Question Asked 3 years. I've read that it's good practice to generate a random key. If I do that, then both the server and the client would need to know the same key. Using aes As System.Security.Cryptography.Aes = System.Security.Cryptography.Aes.Create, unencryptedStream As.
getRandomValues()
 is the only member of the Crypto
 interface which can be used from an insecure context.
Syntax
Parameters
typedArray
- An integer-based
TypedArray
, that is anInt8Array
, aUint8Array
, anInt16Array
, aUint16Array
, anInt32Array
, or aUint32Array
. All elements in the array are overwritten with random numbers.
Return value
The same array passed as typedArray
 but with its contents replaced with the newly generated random numbers. Note that typedArray
is modified in-place, and no copy is made.
Exceptions
This method can throw an exception under error conditions.
QuotaExceededError
- The requested length exceeds 65,536 bytes.
Usage notes
Don't use getRandomValues()
 to generate encryption keys. Instead, use the generateKey()
method. There are a few reasons for this; for example, getRandomValues()
 is not guaranteed to be running in a secure context.
There is no minimum degree of entropy mandated by the Web Cryptography specification. User agents are instead urged to provide the best entropy they can when generating random numbers, using a well-defined, efficient pseudorandom number generator built into the user agent itself, but seeded with values taken from an external source of pseudorandom numbers, such as a platform-specific random number function, the Unix /dev/urandom
 device, or other source of random or pseudorandom data.
Examples
Specification
Specification | Status | Comment |
---|---|---|
Web Cryptography API | Recommendation | Initial definition |
Browser compatibility

Desktop | Mobile | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
Chrome | Edge | Firefox | Internet Explorer | Opera | Safari | Android webview | Chrome for Android | Firefox for Android | Opera for Android | Safari on iOS | Samsung Internet | |
getRandomValues | ChromeFull support 11 | EdgeFull support 12 | FirefoxFull support 26 | IEFull support 11 | OperaFull support 15 | SafariFull support 6.1 | WebView AndroidFull support ≤37 | Chrome AndroidFull support 18 | Firefox AndroidFull support 26 | Opera AndroidFull support 14 | Safari iOSFull support 6.1 | Samsung Internet AndroidFull support 1.0 |
Legend
- Full support Â
- Full support
See also
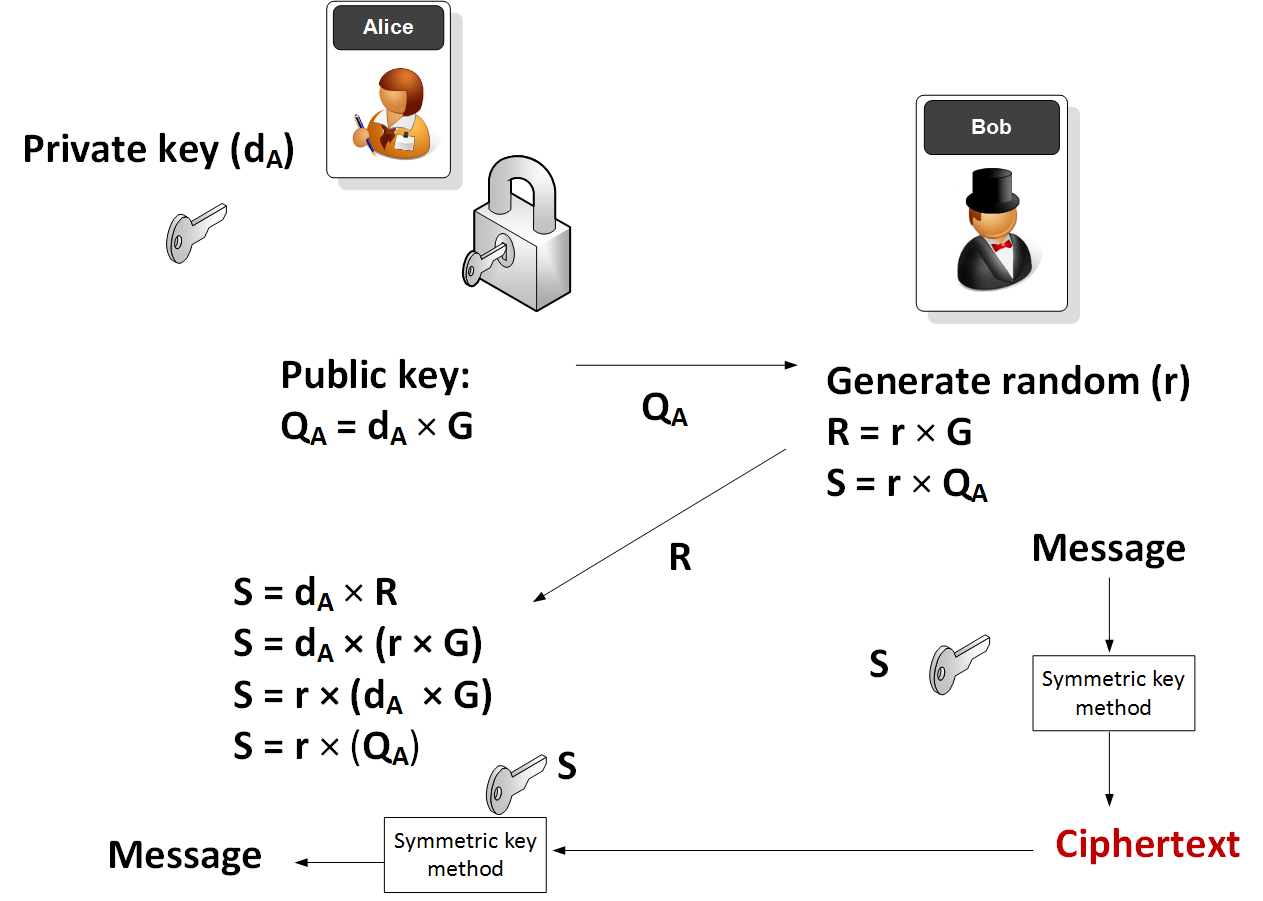
Window.crypto
to get aCrypto
object.Math.random
, a non-cryptographic source of random numbers.
Steam
Definition
Represents the abstract base class from which all implementations of the Advanced Encryption Standard (AES) must inherit.
- Derived
Examples
The following example demonstrates how to encrypt and decrypt sample data by using the Aes class.
Constructors
Aes() | Initializes a new instance of the Aes class. |
Data Security And Cryptography
Fields
BlockSizeValue | Represents the block size, in bits, of the cryptographic operation. (Inherited from SymmetricAlgorithm) |
FeedbackSizeValue | Represents the feedback size, in bits, of the cryptographic operation. (Inherited from SymmetricAlgorithm) |
IVValue | Represents the initialization vector (IV) for the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
KeySizeValue | Represents the size, in bits, of the secret key used by the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
KeyValue | Represents the secret key for the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
LegalBlockSizesValue | Specifies the block sizes, in bits, that are supported by the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
LegalKeySizesValue | Specifies the key sizes, in bits, that are supported by the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
ModeValue | Represents the cipher mode used in the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
PaddingValue | Represents the padding mode used in the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
Properties
Cryptography In Information Security
BlockSize | Gets or sets the block size, in bits, of the cryptographic operation. (Inherited from SymmetricAlgorithm) |
FeedbackSize | Gets or sets the feedback size, in bits, of the cryptographic operation for the Cipher Feedback (CFB) and Output Feedback (OFB) cipher modes. (Inherited from SymmetricAlgorithm) |
IV | Gets or sets the initialization vector (IV) for the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
Key | Gets or sets the secret key for the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
KeySize | Gets or sets the size, in bits, of the secret key used by the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
LegalBlockSizes | Gets the block sizes, in bits, that are supported by the symmetric algorithm. |
LegalKeySizes | Gets the key sizes, in bits, that are supported by the symmetric algorithm. |
Mode | Gets or sets the mode for operation of the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
Padding | Gets or sets the padding mode used in the symmetric algorithm. (Inherited from SymmetricAlgorithm) |
Methods
Clear() | Releases all resources used by the SymmetricAlgorithm class. (Inherited from SymmetricAlgorithm) |
Create() | Creates a cryptographic object that is used to perform the symmetric algorithm. |
Create(String) | Creates a cryptographic object that specifies the implementation of AES to use to perform the symmetric algorithm. |
CreateDecryptor() | Creates a symmetric decryptor object with the current Key property and initialization vector (IV). (Inherited from SymmetricAlgorithm) |
CreateDecryptor(Byte[], Byte[]) | When overridden in a derived class, creates a symmetric decryptor object with the specified Key property and initialization vector (IV). (Inherited from SymmetricAlgorithm) |
CreateEncryptor() | Creates a symmetric encryptor object with the current Key property and initialization vector (IV). (Inherited from SymmetricAlgorithm) |
CreateEncryptor(Byte[], Byte[]) | When overridden in a derived class, creates a symmetric encryptor object with the specified Key property and initialization vector (IV). (Inherited from SymmetricAlgorithm) |
Dispose() | Releases all resources used by the current instance of the SymmetricAlgorithm class. (Inherited from SymmetricAlgorithm) |
Dispose(Boolean) | Releases the unmanaged resources used by the SymmetricAlgorithm and optionally releases the managed resources. (Inherited from SymmetricAlgorithm) |
Equals(Object) | Determines whether the specified object is equal to the current object. (Inherited from Object) |
GenerateIV() | When overridden in a derived class, generates a random initialization vector (IV) to use for the algorithm. (Inherited from SymmetricAlgorithm) |
GenerateKey() | When overridden in a derived class, generates a random key (Key) to use for the algorithm. (Inherited from SymmetricAlgorithm) |
GetHashCode() | Serves as the default hash function. (Inherited from Object) |
GetType() | Gets the Type of the current instance. Trackmania 2 canyon serial key generator new.rar password. (Inherited from Object) |
MemberwiseClone() | Creates a shallow copy of the current Object. (Inherited from Object) |
ToString() | Returns a string that represents the current object. (Inherited from Object) |
ValidKeySize(Int32) | Determines whether the specified key size is valid for the current algorithm. (Inherited from SymmetricAlgorithm) |
Explicit Interface Implementations
System Security Cryptography Algorithms
IDisposable.Dispose() | Releases the unmanaged resources used by the SymmetricAlgorithm and optionally releases the managed resources. (Inherited from SymmetricAlgorithm) |